After two terms of running a weekly course on App Development with Swift, there are thoughts to the curriculum and resources that Apple developed for students to learn about coding in Swift.
Curriculum
The course has two main resources that run hand in hand: the iBook App Development with Swift and its resource files. The student resource files contains Playground files and the book’s source codes together with needed media files for some projects described in the book.
There are also accompanying resources for teachers. The first is the teacher’s guide to the iBook. The second contains answers to the corresponding student’s Playground files.
Book structure
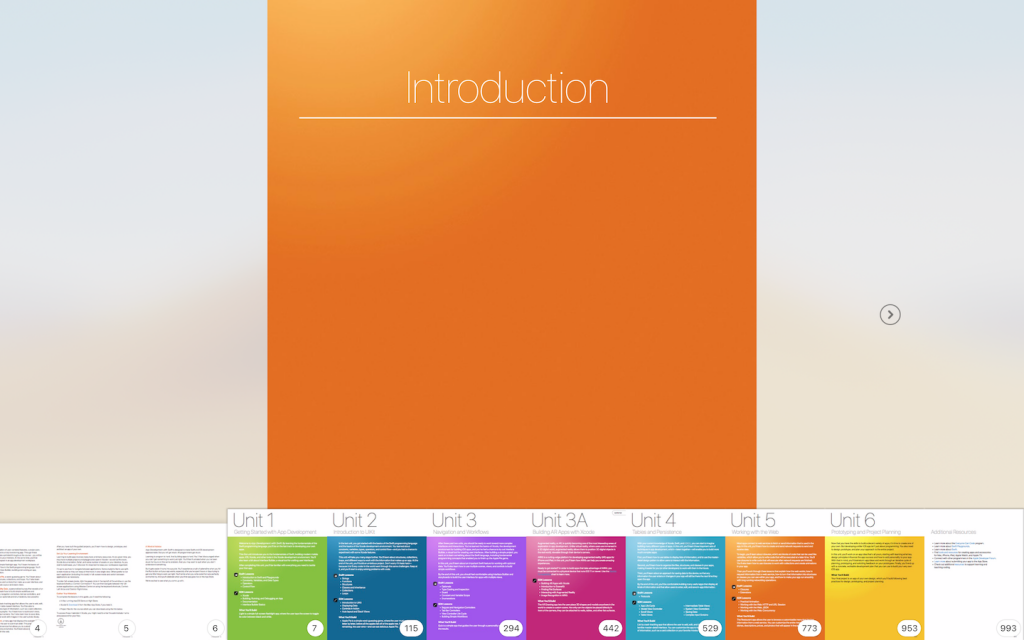
The iBook itself is divided into seven main parts:
- Getting Started with App Development
- Introduction to UIKit
- Navigation and Workflows
- Building AR Apps with Xcode
- Tables and Persistence
- Working with the Web
- Prototyping and Project Planning
Part 1
In part 1, you get to learn the fundamentals of programming, where you were introduced to different data types, starting with String
. Structures were also briefly introduced, although the details would be covered again in Unit 2. Next, Operators are covered before Control Flow, where you would learn about conditionals using if-else
and switch
. The unit ended with ternary operator where you can code an if-else
conditional within a statement.
if a > b {
largest = a
} else {
largest = b
}
The above code could be shortened using a ternary operator:
largest = a > b ? a : b
Students are then introduced to the Xcode environment, including building and running an app.
Unit 1 ended with a guided project to create a torch light app. Students learnt about adding IBOutlet
and IBAction
to the app, and using if
statements to turn on and off the torch light.
Students progress
Students attending my course are generally able to follow the textbook instructions and Playground activities in Unit 1.
One very common error encountered by students is typo errors. At this stage, when students saw error messages, some were able to correct themselves, although error messages could be very misleading. Another common mistake made by students were placing codes in the wrong places. This error is common because students were not used to reading the positions of braces in the textbook codes. A similar problem can happen when using the Interface Builder to create the UI.
Students were very excited the first time they encountered the simulator when running the torch light app. It is appropriate for Apple to introduce the torch light app at the very beginning to bring excitment to students.
Part 2
Part 2 introduces more abstract aspects of programming, namely structures, classes and collections(arrays and dictionaries). The main feedback from students were that they were confused by the instructions in the textbook. Since they had never encountered the concepts of structures, classes and collections, they were confused with the reasons why they were doing what they were told to do in the book, since they have no similar ideas to relate to.
This was the importance of face-to-face sessions, where I can explain the reasons behind their confusions. If left to themselves, many students would have difficulties understanding the instructions. In fact, this is the same type of difficulty when I tried to pick up a new language or framework.
I remember when I was learning programming on Mac OS X and iPhone (back in the days when it was still in Objective-C), I had great difficulties learning from books. It was not so much as following the instructions and typing the code(although typo errors compounded to the learning process), but more with understanding the rationale behind many of the processes. I recalled I was once not able to differentiate between IBAction
and IBOutlet
. It took me a lot of time to re-read the contents over before I got the idea why certain things were done as instructed.
Source control
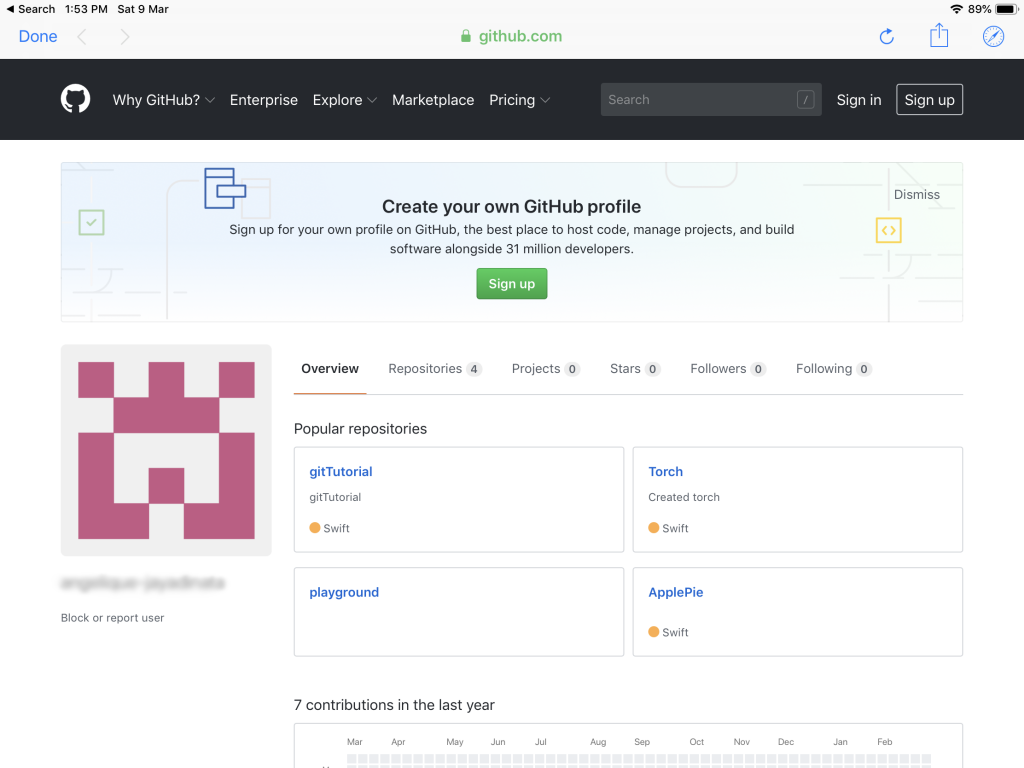
An added feature in my course was the adding of source control with GitHub. The purpose of adding GitHub is
- putting student’s codes online so that I can inspect them, especially if their codes fail to build.
- to introduce them to an important technique of programming that is usually not covered when learning a new language.
- for students to showcase their works when they are preparing for university applications.
With GitHub, I do not need to worry about the repeat emails from students and their codes. To inspect their codes, I just need to access their repo at GitHub.
To create remote repo at GitHub, I just ask the students to type the following commands in Terminal.
git add .
git commit -m "Commit message"
git remote add origin https://github.com/username/project.git
git push origin master
The commands above were used for first time commit to a remote repository. For subsequent push, they just need to add, commit and push, as shown below:
git add .
git commit -m "Commit message"
git push origin
With GitHub firmly in place, students would then start adding codes and UI.
Thoughts
Having taught for two terms, I have some thoughts about the curriculum that Apple developed to teach Swift and apps development for the iOS platform.
First, I think Apple has done a wonderful curriculum to educate programming and app development. Certainly, programming is not easy, let alone app development, which requires additional considerations such as UI.
In fact, Apple does provide an easier course on app development(Intro to App Development with Swift). In that course, it dealt more with creating simple user interface with Interface Builder and linking them up with code. However, it provides too little knowledge for learners who completed it with the needed knowledge to write useful applications. Personally, I feel the this App Development with Swift is much more interesting and useful for students to learn and apply once they completed the course. Moreover, the students I took for the course are high school students, and without any point of reference, I allocated one year, which is also the recommended time line for course completion.
However, being delivered as a co-curricular activity, which is done once a week, excluding holidays and exam periods, I found it hard pressed to complete the required contents. In addition, the students found the concepts confusing, and I concur since they have no previous experiences and ideas to relate such concepts to.
Another point to consider is that after two terms, we have only done single view apps, which is supposedly to be the easiest apps to write. Following this, the course would discuss navigation controllers and table views, which are much harder to understand. To add to this, additional topics such as AR(Augmented Reality) and Web are also included.
With these considerations, it would be quite difficult to complete the entire course within a single year. In order to do so, there are some possible solutions.
Topics coverage
Many topics covered in the book are important, and I do not think it is possible to remove them if we want the students to write useful meaningful apps at the end of the course. Topics like navigation controllers, tableviews and persistence are too important to skipped.
However, two topics currently in the book could be reserved for more advanced or niche topic for further studies. As this course was intended to introduce app development to students without prerequisites, AR and Web could be skipped. Augmented Reality, although it is a current hot topic, has niche use. Even at this point in time, there are little apps in the App Store that uses AR. On the other hand, although interfacing with a web app is a commonly used feature, such techniques could still be reserved for a further advanced course.
Possible ways to improve the course
I think source control is important and should be included. Learning app development is a complicated business. It is common for someone to reach a certain successful stage following a book, before suddenly finding that his app fails to build. If source control is taught, learners could revert to previous successful stage and continue.
Additionally, by pushing their codes to remote repository, course trainer may review the codes consistently and provide feedback to the students. Students may also use the repository as evidence that they have done coding projects.
Errors are common in coding. The book could introduce more about debugging techniques, including setting breakpoints and running through the code step by step.
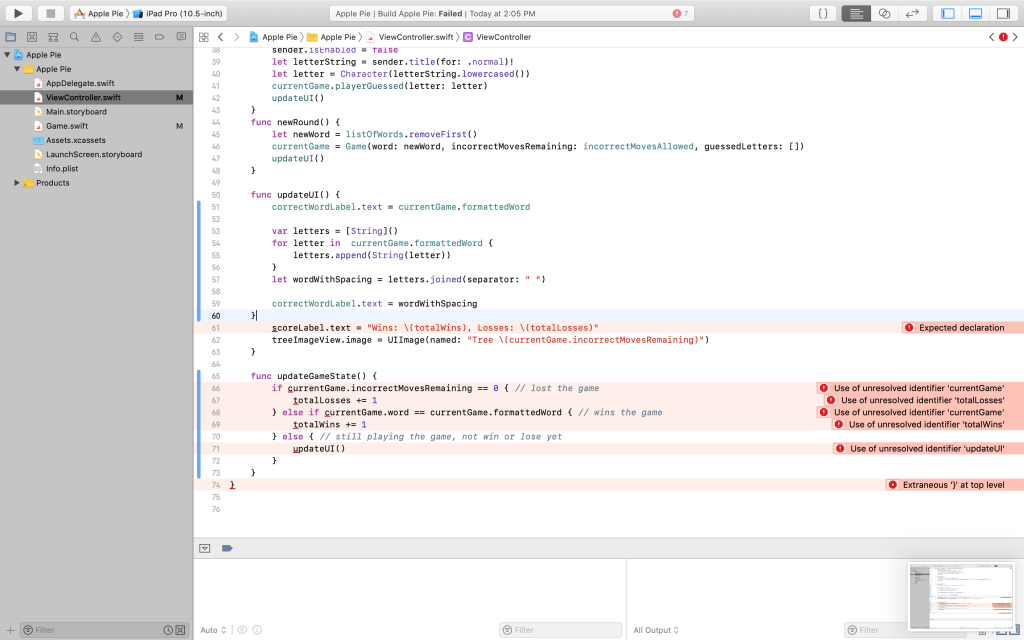
Lastly, one can never learn everything about the framework. The course should integrate documentation reference regularly so that students could extend framework knowledge by themselves. This skill allows them to also develop more creative apps besides what was taught in the book.
Conclusion
To conclude, I think the course is great. It provides numerous resources such as the iBook and practice exercises in the form of Playgrounds. It even provide a teacher’s solution for instructors to refer to.
The content coverage is comprehensive. Coding is hard, and one should not try to water down the difficulty, as regularly seen on coding sites for learners that does all the backend work and requires the learner to just enter a function name to see magic. Coding is not magic. Those sites, such as Hour of Code, are good for exposure, but provide little use in terms of teaching proper coding skills. That being said, it is important to focus the learner to only a specific set of basic skills so as not to confuse the learner.
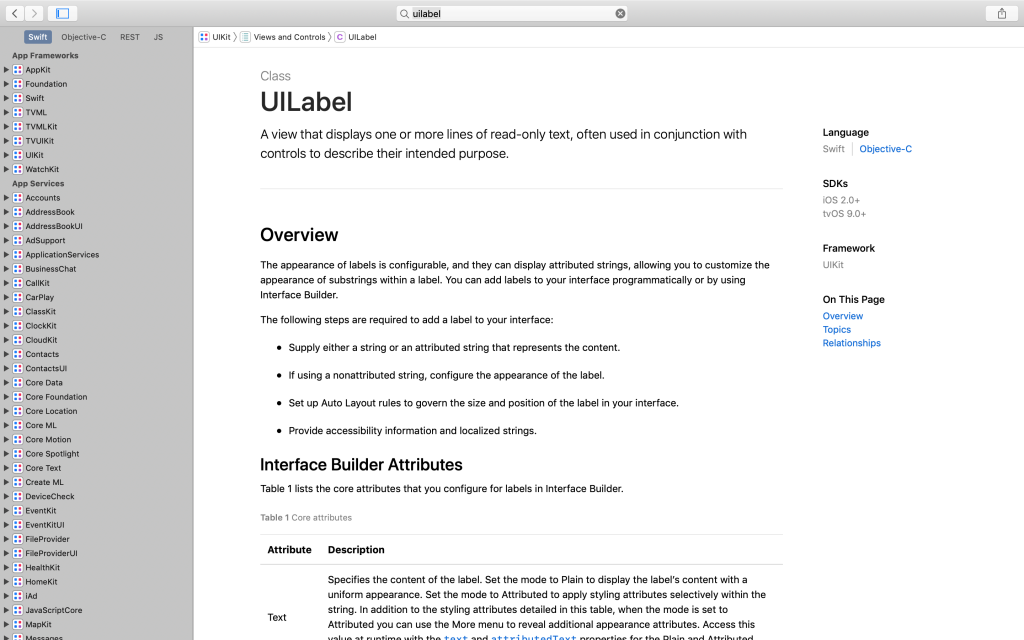
However, certain important techniques that are commonly used by software engineers should also be introduced in the book. Techniques such as debugging, source control and documentation reference help students to venture further than what was taught in the book. Such topics, replacing AR and Web, could make the curriculum more extensible.